Daten-IO
Contents
Daten-IO#
Daten laden und speichern
NumPy Befehle#
Link: NumPy Input and output
import numpy as np
import matplotlib.pyplot as plt
Daten aus Textdatei einlesen:
A = np.genfromtxt('daten/AustriaW-o.csv', delimiter=",", skip_header=1)
A
array([[ 47.235154 , 9.59921836, 467. ],
[ 47.23515 , 9.59921 , 466. ],
[ 47.23451 , 9.59925 , 465. ],
...,
[ 47.94042 , 17.06803 , 142. ],
[ 47.94034 , 17.06798 , 142. ],
[ 47.94011 , 17.06859 , 140. ]])
elevation = A[:,2]
plt.figure(figsize=(8,3))
plt.plot(elevation)
plt.xlabel('Datenpunkt')
plt.ylabel('elevation')
plt.grid(True)
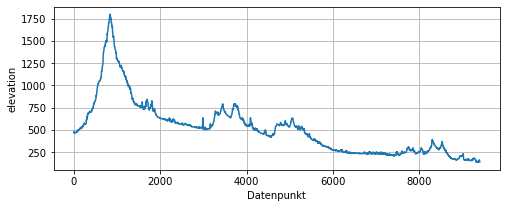
Matrix von Zufallszahlen:
B = np.random.randn(10, 3)
B
array([[ 1.47968301, 0.7744056 , -1.56402179],
[-1.34156172, 0.04479663, -0.09928773],
[ 2.02129895, -1.03331223, -0.54331106],
[ 0.92777002, 1.09598418, 0.51193523],
[ 1.6080908 , -1.17283388, -0.57101294],
[ 1.01146909, 0.25710312, -0.06671634],
[-0.51868207, 1.10980157, -1.46367182],
[-0.82497953, 0.52637745, -0.21615519],
[-0.01972374, 0.6880444 , 0.93750325],
[-1.00788025, 0.88291008, 0.53424519]])
Speichern als CSV-Datei:
np.savetxt('daten/Zufallszahlenmatrix.csv', B, delimiter=',')
Pickle#
Speichern und Laden von Objekten jeglichen Datentyps. Siehe z. B. wiki.python.org/moin/UsingPickle
import pickle
my_object = ['Ich', 'und', 7]
pickle.dump( my_object, open( "daten/save.p", "wb" ) )
del my_object
%whos
Variable Type Data/Info
--------------------------------
A ndarray 9398x3: 28194 elems, type `float64`, 225552 bytes (220.265625 kb)
B ndarray 10x3: 30 elems, type `float64`, 240 bytes
elevation ndarray 9398: 9398 elems, type `float64`, 75184 bytes
np module <module 'numpy' from '/us<...>kages/numpy/__init__.py'>
pickle module <module 'pickle' from '/u<...>ib/python3.10/pickle.py'>
plt module <module 'matplotlib.pyplo<...>es/matplotlib/pyplot.py'>
my_object = pickle.load( open( "daten/save.p", "rb" ) )
my_object
['Ich', 'und', 7]